Structured Query Language (SQL) is a powerful tool used for managing and manipulating relational databases. This blog post delves into the intricacies of SQL, its various types, and provides detailed explanations and examples.
Introduction
SQL, or Structured Query Language, is the standard language for interacting with relational databases. Whether you’re querying data, updating records, or managing database structures, SQL is the tool for the job. Its importance in today’s data-driven world cannot be overstated, as it underpins a wide array of applications, from small personal projects to large-scale enterprise systems.
What is SQL?
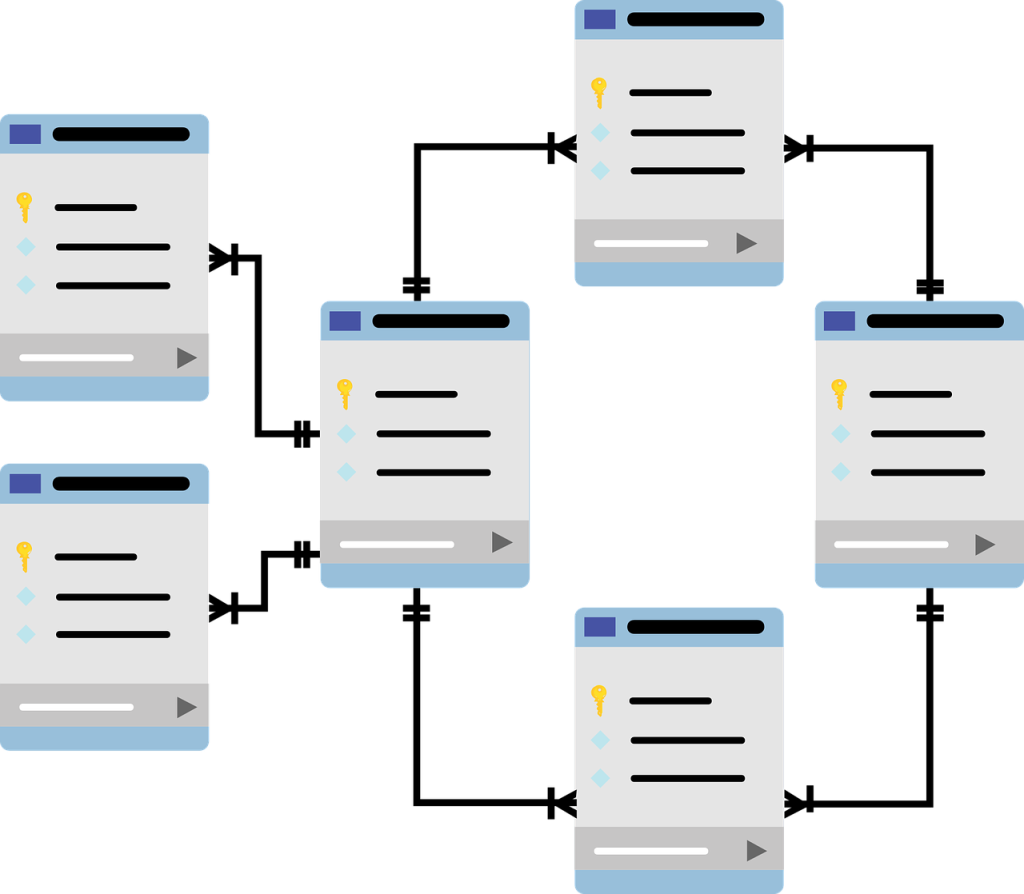
SQL stands for Structured Query Language. It’s used to communicate with and manipulate databases. SQL was initially developed at IBM in the early 1970s and has since become the standard language for relational database management systems (RDBMS).
Basic Concepts of SQL
SQL operates on tables within databases, where data is stored in rows and columns. Here are some foundational concepts:
- Tables: Structures that hold data in rows and columns.
- Rows: Individual records in a table.
- Columns: Attributes of the data, defining the type of data each row can hold.
Example:
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
HireDate DATE
);
Output: This command creates a table named Employees
with four columns.
Types of SQL Commands
SQL commands are divided into several categories:
Data Query Language (DQL)
SELECT is used to query data from a database.
SELECT FirstName, LastName FROM Employees WHERE HireDate > '2023-01-01';
Output: A list of employees hired after January 1, 2023.
Data Definition Language (DDL)
Commands that define the structure of a database.
CREATE: Creates a new table.
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(100)
);
Output: A new table Departments
is created.
ALTER: Modifies an existing table.
ALTER TABLE Employees ADD COLUMN DepartmentID INT;
Output: Adds a DepartmentID
column to the Employees
table.
DROP: Deletes a table.
DROP TABLE Departments;
Output: The Departments
table is deleted.
TRUNCATE: Removes all records from a table, but not the table itself.
TRUNCATE TABLE Employees;
Output: All records in the Employees
table are deleted.
Data Manipulation Language (DML)
Commands for modifying data.
INSERT: Adds new records.
INSERT INTO Employees (EmployeeID, FirstName, LastName, HireDate)
VALUES (1, 'John', 'Doe', '2023-06-01');
Output: A new record is added to the Employees
table.
UPDATE: Modifies existing records.
UPDATE Employees SET LastName = 'Smith' WHERE EmployeeID = 1;
Output: The last name of the employee with EmployeeID
1 is updated to ‘Smith’.
DELETE: Removes records.
DELETE FROM Employees WHERE EmployeeID = 1;
Output: The record with EmployeeID
1 is deleted.
Data Control Language (DCL)
Commands for managing access to the database.
GRANT: Gives permissions to users.
GRANT SELECT, INSERT ON Employees TO 'user1';
Output: User user1
is granted permission to select and insert data in the Employees
table.
REVOKE: Removes permissions from users.
REVOKE INSERT ON Employees FROM 'user1';
Output: User user1
no longer has permission to insert data in the Employees
table.
Transaction Control Language (TCL)
Commands for managing transactions.
COMMIT: Saves changes made during the transaction.
COMMIT;
Output: All changes made during the transaction are saved.
ROLLBACK: Reverts changes made during the transaction.
ROLLBACK;
Output: All changes made during the transaction are undone.
SAVEPOINT: Sets a point within a transaction to which you can rollback.
SAVEPOINT Savepoint1;
Output: A savepoint named Savepoint1
is created.
Advanced SQL Features
SQL offers advanced features for complex queries and data manipulation.
Joins
Joins combine rows from two or more tables based on related columns.
INNER JOIN: Returns records with matching values in both tables.
SELECT Employees.FirstName, Employees.LastName, Departments.DepartmentName
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Output: A list of employees with their respective department names.
LEFT JOIN: Returns all records from the left table and matched records from the right table.
SELECT Employees.FirstName, Employees.LastName, Departments.DepartmentName
FROM Employees
LEFT JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Output: All employees and their department names, with NULLs for employees without a department.
RIGHT JOIN: Returns all records from the right table and matched records from the left table.
SELECT Employees.FirstName, Employees.LastName, Departments.DepartmentName
FROM Employees
RIGHT JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Output: All departments and their employees, with NULLs for departments without employees.
FULL JOIN: Returns all records when there is a match in either table.
SELECT Employees.FirstName, Employees.LastName, Departments.DepartmentName
FROM Employees
FULL JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Output: All employees and departments, with NULLs where there is no match.
Subqueries and Nested Queries
Queries within queries to perform complex operations.
SELECT FirstName, LastName
FROM Employees
WHERE DepartmentID = (SELECT DepartmentID FROM Departments WHERE DepartmentName = 'HR');
Output: Employees who belong to the ‘HR’ department.
Indexes and Performance Tuning
Indexes improve the speed of data retrieval.
CREATE INDEX idx_lastname ON Employees (LastName);
Output: An index on the LastName
column to speed up search queries.
Views
Virtual tables created by a query.
CREATE VIEW EmployeeView AS
SELECT FirstName, LastName, DepartmentName
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Output: A view named EmployeeView
that combines employee and department data.
Stored Procedures and Functions
Reusable SQL code for complex operations.
Stored Procedure:
CREATE PROCEDURE GetEmployeesByDepartment (IN deptName VARCHAR(100))
BEGIN
SELECT FirstName, LastName FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID
WHERE Departments.DepartmentName = deptName;
END;
Output: A stored procedure to get employees by department.
Function:
CREATE FUNCTION CalculateAge (dob DATE) RETURNS INT
BEGIN
DECLARE age INT;
SET age = TIMESTAMPDIFF(YEAR, dob, CURDATE());
RETURN age;
END;
Output: A function to calculate age from date of birth.
Triggers
Automatic actions executed when certain events occur.
CREATE TRIGGER BeforeInsertEmployee
BEFORE INSERT ON Employees
FOR EACH ROW
BEGIN
SET NEW.HireDate = IFNULL(NEW.HireDate, CURDATE());
END;
Output: A trigger to set the hire date to the current date if none is provided.
SQL in Different Database Systems
SQL syntax varies slightly between different database systems.
MySQL:
SELECT NOW();
Output: Current date and time in MySQL.
PostgreSQL:
SELECT CURRENT_TIMESTAMP;
Output: Current date and time in PostgreSQL.
Microsoft SQL Server:
SELECT GETDATE();
Output: Current date and time in SQL Server.
Oracle Database:
SELECT SYSDATE FROM DUAL;
Output: Current date and time in Oracle.
Practical Applications of SQL
SQL is used in various fields for data analysis, business intelligence, and web development.
Data Analysis:
SELECT DepartmentID, COUNT(*) as EmployeeCount
FROM Employees
GROUP BY DepartmentID;
Output: Number of employees in each department.
Business Intelligence:
SELECT AVG(Salary) as AverageSalary
FROM Employees
WHERE DepartmentID = (SELECT DepartmentID FROM Departments WHERE DepartmentName = 'IT');
Output: Average salary of employees in the ‘IT’ department.
Web Development:
SQL is used to interact with databases in web applications.
Common SQL Challenges and Best Practices
Handling large datasets, ensuring security, and optimizing queries are common challenges in SQL.
Handling Large Datasets
Use indexing and partitioning to manage large datasets efficiently.
Security Considerations
Ensure proper user permissions and use prepared statements to prevent SQL injection.
Optimizing SQL Queries
Analyze query execution plans and optimize indexes and query structures.
Debugging and Error Handling
Use error handling mechanisms in stored procedures and functions.
Learning and Mastering SQL
Resources for learning SQL include online courses, tutorials, and books. Practice regularly and explore advanced topics.
Resources:
- Online platforms like Coursera, Udemy, and Khan Academy.
- Books like “SQL For Dummies” and “Learning SQL”.
Future of SQL
SQL continues to evolve with trends like SQL on Hadoop, integration with machine learning, and the rise of NewSQL databases.
SQL vs. NoSQL
SQL databases are structured and suitable for complex queries, while NoSQL databases are flexible and handle unstructured data well.
Conclusion
SQL remains a cornerstone of data management and manipulation, serving as a versatile and powerful tool for a wide range of applications. Its structured nature and robust querying capabilities make it indispensable for tasks ranging from simple data retrieval to complex analytics and reporting. As we continue to generate and rely on vast amounts of data, the ability to efficiently interact with databases using SQL will remain a critical skill.
Moreover, the ongoing evolution of SQL, with new features and integrations, ensures that it stays relevant in an ever-changing technological landscape. Whether you are a novice beginning your journey in data management or an experienced professional looking to deepen your expertise, mastering SQL opens up numerous opportunities in fields such as data analysis, business intelligence, software development, and beyond.
In a world where data drives decisions and innovation, SQL stands out as a fundamental skill that empowers individuals and organizations to harness the full potential of their data. Embracing SQL is not just about learning a language; it’s about unlocking the ability to turn data into actionable insights and competitive advantage.