Introduction to PHP
Definition and Brief History
PHP, an acronym for Hypertext Preprocessor, is a widely-used open-source scripting language specifically designed for web development. It was created by Rasmus Lerdorf in 1994 and initially stood for “Personal Home Page” tools. However, as the language evolved, it was renamed to PHP: Hypertext Preprocessor. PHP scripts are executed on the server, generating HTML which is then sent to the client. This server-side processing capability allows for dynamic content generation, making PHP a crucial tool in modern web development.
PHP’s journey began modestly when Lerdorf wrote several Common Gateway Interface (CGI) programs in C to maintain his personal homepage. The simplicity and effectiveness of these scripts quickly garnered attention from the developer community. By 1997, PHP/FI 2.0 was released, and it was estimated that around 50,000 domains were using PHP. The major turning point came with PHP 3, which was a complete rewrite of the language and marked the beginning of PHP’s widespread adoption.
The Creator: Rasmus Lerdorf
Rasmus Lerdorf, a Danish-Canadian programmer, developed PHP to manage his personal website. His initial goal was to track visits to his online resume. However, recognizing the potential of his creation, he released the source code to the public. This decision was instrumental in PHP’s growth, as it allowed developers from around the world to contribute to its development. Lerdorf’s vision of an open-source, accessible scripting language laid the foundation for PHP’s success.
Evolution of PHP Versions
PHP has undergone significant changes since its inception. Each major version brought substantial improvements in functionality, performance, and security.
- PHP 3: Introduced in 1998, PHP 3 marked the beginning of PHP’s growth phase. It introduced several key features, including support for multiple databases and the ability to embed PHP within HTML.
- PHP 4: Released in 2000, PHP 4 offered improved performance and introduced the Zend Engine 1.0, which significantly enhanced the language’s execution speed.
- PHP 5: Launched in 2004, PHP 5 brought robust object-oriented programming (OOP) support, making it a more powerful and flexible language. It also introduced the Zend Engine II, which provided further performance enhancements.
- PHP 7: Released in 2015, PHP 7 was a game-changer. It provided a significant performance boost, with improvements in speed and reduced memory usage. The new Zend Engine (version 3.0) allowed for more efficient execution.
- PHP 8: Introduced in 2020, PHP 8 brought a host of new features such as the Just-In-Time (JIT) compiler, union types, and improvements in type safety. These enhancements further solidified PHP’s position as a leading web development language.
PHP Basics
Syntax and Structure
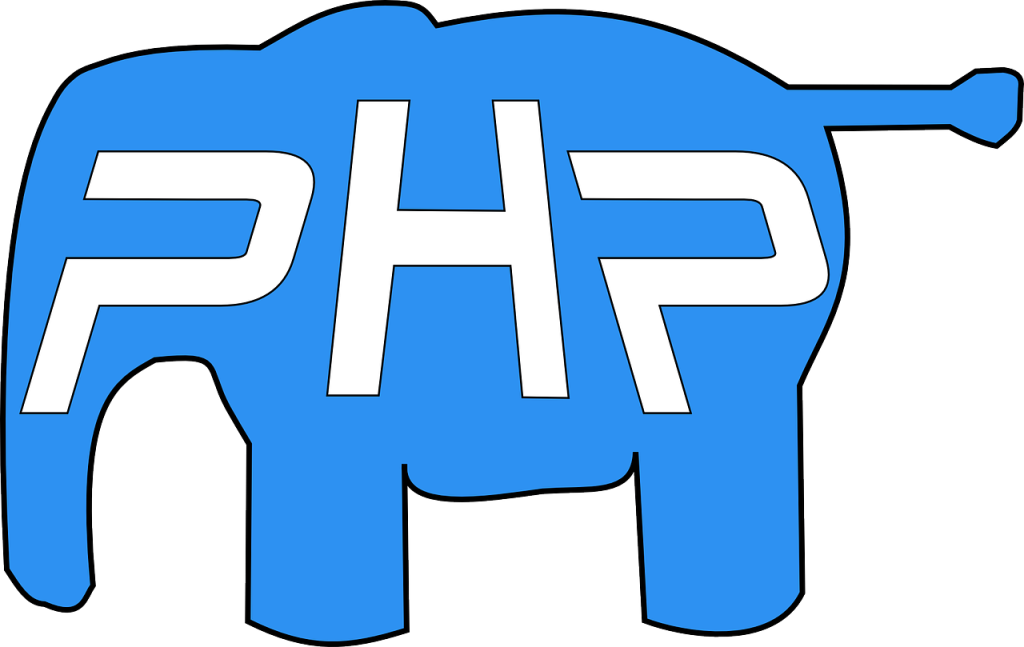
PHP scripts are executed on the server, and the result is returned to the browser as plain HTML. This server-side processing allows for the creation of dynamic and interactive web pages. A basic PHP script is embedded within HTML using the <?php ... ?>
tags. Here’s an example of a simple PHP script:
<?php
echo "Hello, World!";
?>
In this example, the echo
statement outputs “Hello, World!” to the web page. PHP code can be placed anywhere within the HTML, and the server will process it before sending the output to the client.
Variables, Constants, and Data Types
PHP supports a variety of data types, including integers, floats, strings, arrays, and objects. Variables in PHP are declared with a $
sign followed by the variable name. Constants are defined using the define()
function. Here’s an example:
<?php
$greeting = "Hello, World!";
define("SITE_NAME", "Learncooding");
echo $greeting; // Outputs: Hello, World!
echo SITE_NAME; // Outputs: Learncooding
?>
PHP is a loosely typed language, meaning that variables do not need to be declared with a specific data type. The language automatically converts the variable to the correct data type based on its value.
Basic PHP Commands and Functions
PHP offers a wide range of built-in functions to perform various tasks. Some fundamental commands include:
echo
andprint
: Used for outputting data to the web page.include
andrequire
: Used to include files within a PHP script. The difference is thatrequire
will produce a fatal error and stop the script if the file is not found, whileinclude
will only produce a warning and continue execution.isset()
andempty()
: Used to check variable states.isset()
determines if a variable is set and is not null, whileempty()
checks if a variable is empty.
Here’s an example demonstrating these commands:
<?php
$number = 42;
$text = "Learncooding";
if (isset($number)) {
echo "The variable is set.";
}
if (empty($text)) {
echo "The variable is empty.";
} else {
echo "The variable is not empty.";
}
include 'footer.php';
?>
The Rise of PHP
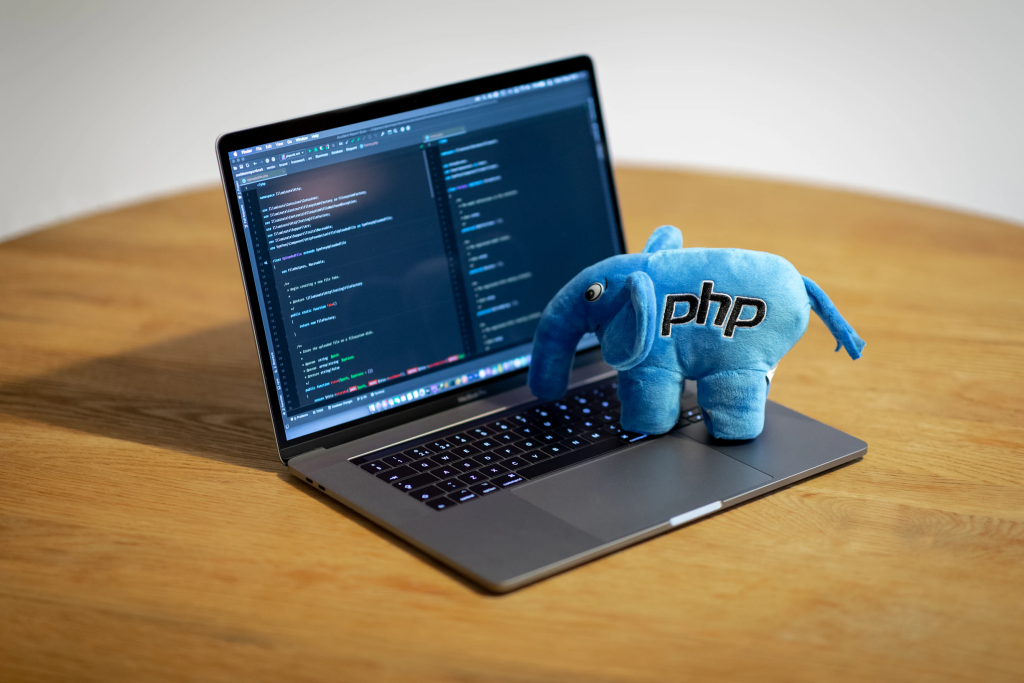
Early Adoption and Community Support
PHP’s early adoption was driven by its simplicity and ability to integrate seamlessly with HTML. This made it an attractive choice for web developers looking to create dynamic websites quickly. As more developers started using PHP, a vibrant and active community formed around it. This community played a crucial role in PHP’s growth, contributing to its development and creating a rich ecosystem of tools and resources.
Integration with HTML and Web Development
One of PHP’s key strengths is its ability to be embedded directly into HTML. This integration allows developers to create dynamic web pages without the need for additional complex configurations. Here’s an example of PHP embedded in HTML:
<!DOCTYPE html>
<html>
<head>
<title>Welcome to Learncooding</title>
</head>
<body>
<h1><?php echo "Hello, World!"; ?></h1>
<p>Welcome to our website!</p>
</body>
</html>
In this example, the PHP code <?php echo "Hello, World!"; ?>
is embedded within the HTML, demonstrating how PHP can be used to generate dynamic content.
PHP and the LAMP Stack
PHP is a core component of the LAMP stack, which stands for Linux, Apache, MySQL, and PHP. This stack provides a powerful and flexible environment for developing and deploying web applications. Each component of the LAMP stack plays a crucial role:
- Linux: The operating system that provides the foundation.
- Apache: The web server that handles requests and serves web pages.
- MySQL: The database management system used for storing and retrieving data.
- PHP: The server-side scripting language used for generating dynamic content.
The LAMP stack’s open-source nature and extensive community support make it a popular choice for web developers.
Features and Advantages of PHP
Open Source Nature
One of the key advantages of PHP is its open-source nature. This means that PHP is free to use, and its source code is available for anyone to inspect, modify, and enhance. The open-source community has played a significant role in PHP’s development, contributing countless libraries, frameworks, and tools that extend its capabilities.
Ease of Learning and Use
PHP’s user-friendly syntax makes it an excellent choice for beginners. The language is easy to understand and write, allowing new developers to quickly grasp the basics and start creating dynamic web pages. Additionally, PHP’s extensive documentation and community resources provide ample support for learners.
Flexibility and Versatility
PHP is a highly flexible and versatile language, capable of handling a wide range of tasks. It can be used for everything from simple scripts to complex web applications. PHP supports various databases, including MySQL, PostgreSQL, and SQLite, making it a flexible solution for different project requirements.
Wide Range of Frameworks and Libraries
PHP has a rich ecosystem of frameworks and libraries that streamline development and enhance productivity. Some of the most popular PHP frameworks include:
- Laravel: Known for its elegant syntax and comprehensive feature set, Laravel simplifies common web development tasks such as routing, authentication, and session management.
- Symfony: A robust framework that provides reusable components and tools for building complex web applications.
- CodeIgniter: A lightweight framework known for its speed and simplicity, making it ideal for small to medium-sized projects.
These frameworks provide developers with a structured foundation, reducing development time and effort.
Performance and Scalability
Optimized for Web Applications
PHP is optimized for creating web applications, making it fast and efficient. The language is designed to handle a large number of users and requests simultaneously, which is crucial for web applications that need to scale.
Handling High Traffic Websites
Many high-traffic websites rely on PHP due to its ability to manage substantial user loads efficiently. For example, Facebook, one of the world’s largest social networking platforms, was initially developed using PHP and continues to use it for many features. Other notable websites like Wikipedia and WordPress also rely on PHP for their operations.
Comparison with Other Languages
PHP often gets compared to other server-side languages like ASP.NET and Ruby on Rails. While each language has its strengths, PHP’s simplicity, extensive community support, and rich feature set make it a preferred choice for many developers. Here’s a brief comparison:
- ASP.NET: A framework developed by Microsoft, ASP.NET is known for its robust performance and integration with other Microsoft products. However, it typically requires a Windows server environment, which can be a limitation for some developers.
- Ruby on Rails: Known for its elegant syntax and convention over configuration approach, Ruby on Rails is a popular framework for web development. However, it has a steeper learning curve compared to PHP.
Community and Ecosystem
Strong Community Support
The PHP community is one of its greatest strengths. Developers worldwide contribute to PHP’s continuous improvement, sharing code, knowledge, and resources. This collaborative environment fosters innovation and ensures that PHP remains up-to-date with the latest web development trends.
Abundance of Learning Resources
There are numerous tutorials, forums, and documentation available for PHP learners. Websites like Learncooding provide extensive resources to help new and experienced developers alike. Whether you’re looking for beginner tutorials or advanced programming guides, the PHP community has you covered.
Contribution to Open Source Projects
PHP’s open-source nature encourages developers to contribute to various projects, fostering innovation and collaboration within the community. Many popular open-source projects, such as WordPress and Magento, are built on PHP, and they benefit from the collective efforts of developers worldwide.
PHP in Modern Web Development
Popular Websites Built with PHP
Many of the world’s leading websites are built with PHP. Here are a few notable examples:
- Facebook: Initially developed using PHP, Facebook continues to use it for many features and backend operations.
- WordPress: The most popular content management system, WordPress powers over 40% of all websites on the internet. Its flexibility and ease of use have made it a favorite among bloggers, businesses, and developers.
- Wikipedia: An extensive online encyclopedia that relies on PHP for its functionality. Wikipedia’s open-source MediaWiki software, which powers the site, is written in PHP.
Use in Content Management Systems
PHP is the backbone of many content management systems (CMS), such as WordPress, Drupal, and Joomla. These CMS platforms have revolutionized web development by allowing users to create and manage websites without needing extensive programming knowledge. Here’s a brief overview of each:
- WordPress: Known for its ease of use and extensive plugin ecosystem, WordPress is the go-to CMS for bloggers and small businesses.
- Drupal: A more advanced CMS that offers greater flexibility and customization options, making it suitable for complex websites and applications.
- Joomla: A user-friendly CMS that balances ease of use and flexibility, making it a popular choice for a wide range of websites.
E-commerce Platforms
PHP powers many e-commerce platforms, providing robust solutions for online businesses. Notable e-commerce platforms built with PHP include:
- Magento: A powerful e-commerce platform known for its flexibility and scalability. Magento offers a wide range of features for managing online stores, including product management, payment processing, and customer relationship management.
- WooCommerce: A popular WordPress plugin that transforms a WordPress site into a fully functional online store. WooCommerce is known for its ease of use and extensive customization options.
The Future of PHP
Current Trends and Updates
PHP continues to evolve with regular updates and new features. PHP 8, for instance, introduced the Just-In-Time (JIT) compiler, which significantly improves performance by compiling code into machine language at runtime. Other notable features in PHP 8 include union types, named arguments, and improvements in error handling.
Ongoing Developments and Innovations
The PHP community is constantly innovating, ensuring the language remains relevant and efficient in the ever-changing tech landscape. Ongoing developments include enhancements to the core language, new frameworks and libraries, and improvements in performance and security.
Challenges and Criticisms
While PHP is powerful, it has faced criticism for security vulnerabilities and inconsistent design. However, with proper coding practices and the use of modern frameworks, these risks can be mitigated.
Security Concerns
PHP’s flexibility and ease of use have led to its widespread adoption, but they have also resulted in security concerns. Common security issues include SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). To address these issues, developers must follow best practices for secure coding and leverage PHP’s built-in security features.
Competition from Emerging Languages
Newer languages and technologies pose a challenge to PHP’s dominance. Languages like Python and JavaScript (with Node.js) have gained popularity for web development. However, PHP’s continuous evolution and vast ecosystem help maintain its position in the industry.
PHP’s Role in the Future of Web Development
Adapting to New Technologies
PHP is adapting to new technologies like cloud computing, the Internet of Things (IoT), and artificial intelligence (AI). These advancements ensure that PHP remains relevant in modern web development. For instance, PHP’s integration with cloud platforms like AWS and Google Cloud allows developers to build scalable and robust applications. PHP frameworks and libraries are also evolving to support new paradigms and technologies, ensuring that developers can leverage PHP for cutting-edge projects.
Potential for Growth and Sustained Popularity
Despite the rise of newer languages, PHP’s potential for growth and sustained popularity remains strong. The language continues to evolve, and the community remains active and innovative. PHP’s adaptability and extensive ecosystem of tools and resources ensure that it will continue to be a valuable asset for web developers.
Conclusion
Summary of Key Points
PHP has come a long way since its inception, evolving into a powerful and versatile language that remains a cornerstone of web development. Its simplicity, community support, and continuous improvements ensure its place in the future of web development. PHP’s integration with HTML, its role in the LAMP stack, and its extensive frameworks and libraries make it a robust choice for developers.